In most applications, maps are one of the most useful tools for users when included in an application. We can easily add a leaflet map in Ionic angular project. The Leaflet is a widely used open-source JavaScript library used to build web mapping applications. It is one of the most popular JavaScript mapping libraries and is used by major websites such as FourSquare, Pinterest, and Flickr.
In this article, we are learning how to add Leaflet map library in the Ionic Angular project and we have demonstrated a few examples along with map marker.
Prerequisites:
To create and run an Ionic project, we need Nodejs and Ionic CLI already install in our system, if not then check how to install Nodejs and Ionic in Ubuntu. Ionic CLI allows us to create an Ionic project, generate application and library code, and perform a variety of ongoing development tasks such as testing, bundling, and deployment. To install the Ionic CLI, open a terminal, and run the following command:
npm install -g @ionic/cli
How to install and configure leaflet map in Ionic 6 angular.?
Let’s create a project to demonstrate an example of how to add a leaflet map in the Ionic 5 projects.
ionic start leafletIonic tabs --type=angular
npm install leaflet --save
npm install leaflet-ant-path --save
Step 2: Configure Leaflet map in ionic project
We have set a leaflet map in an ionic project. For the leaflet map to render correctly, we need to import the Leaflet stylesheet to the Angular project. To do this import leaflet style at the end in global.scss
@import "~leaflet/dist/leaflet.css"
Step 3: Set up the tab template.
We will rename the tab default name to leaflets, and edit app/tabs/tabs.page.html. First, we will display a leaflet map with an ant path between two locations. On the second tab, we will display the different locations and different leaflet map designs. On the last tab, we will display a leaflet map with multiple markers.
<ion-tabs>
<ion-tab-bar slot="bottom">
<ion-tab-button tab="tab1">
<ion-icon name="triangle"></ion-icon>
<ion-label>Leaflet 1 with ant path</ion-label>
</ion-tab-button>
<ion-tab-button tab="tab2">
<ion-icon name="ellipse"></ion-icon>
<ion-label>Leaftlet 2</ion-label>
</ion-tab-button>
<ion-tab-button tab="tab3">
<ion-icon name="square"></ion-icon>
<ion-label>Leaflet with multiple marker</ion-label>
</ion-tab-button>
</ion-tab-bar>
</ion-tabs>
Step 3: Set up First Leaflet map design
In the map method of the leaflet map in ionic, we pass the div id of our map, which we name as a mapId. In our example, we want to center our map on Delhi the capital of India and located at 28.644800 latitudes and longitude 77.216721. We have used a leaflet ant path to draw an animated path from Delhi to Leh.
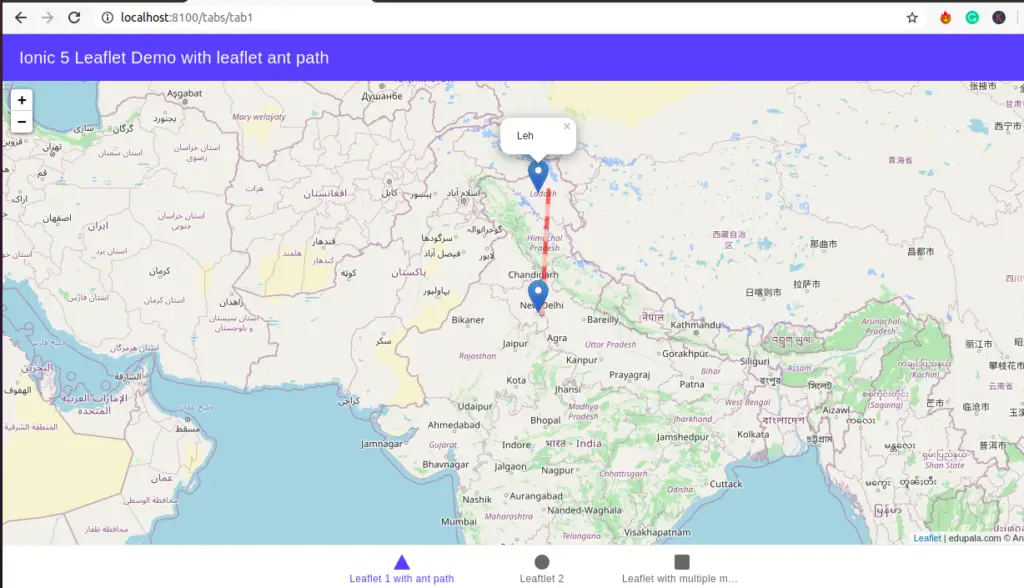
Edit template and typescript component of tab 1 to add our leaflet map with leaflet ant path between two locations.
<ion-header [translucent]="true">
<ion-toolbar color="primary">
<ion-title>
Ionic 5 Leaflet Demo with leaflet ant path
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<div id="mapId" style="width: 100%; height: 100%">
</div>
</ion-content>
import { Component, OnInit, OnDestroy } from '@angular/core';
import * as Leaflet from 'leaflet';
import { antPath } from 'leaflet-ant-path';
@Component({
selector: 'app-tab1',
templateUrl: 'tab1.page.html',
styleUrls: ['tab1.page.scss']
})
export class Tab1Page implements OnInit, OnDestroy {
map: Leaflet.Map;
constructor() { }
ngOnInit() { }
ionViewDidEnter() { this.leafletMap(); }
leafletMap() {
this.map = Leaflet.map('mapId').setView([28.644800, 77.216721], 5);
Leaflet.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: 'edupala.com © Angular LeafLet',
}).addTo(this.map);
Leaflet.marker([28.6, 77]).addTo(this.map).bindPopup('Delhi').openPopup();
Leaflet.marker([34, 77]).addTo(this.map).bindPopup('Leh').openPopup();
antPath([[28.644800, 77.216721], [34.1526, 77.5771]],
{ color: '#FF0000', weight: 5, opacity: 0.6 })
.addTo(this.map);
}
/** Remove map when we have multiple map object */
ngOnDestroy() {
this.map.remove();
}
}
Step 4: Set up Second Leaflet design.
Edit template and typescript of a component of tab2 to add a leaflet map with different designs.
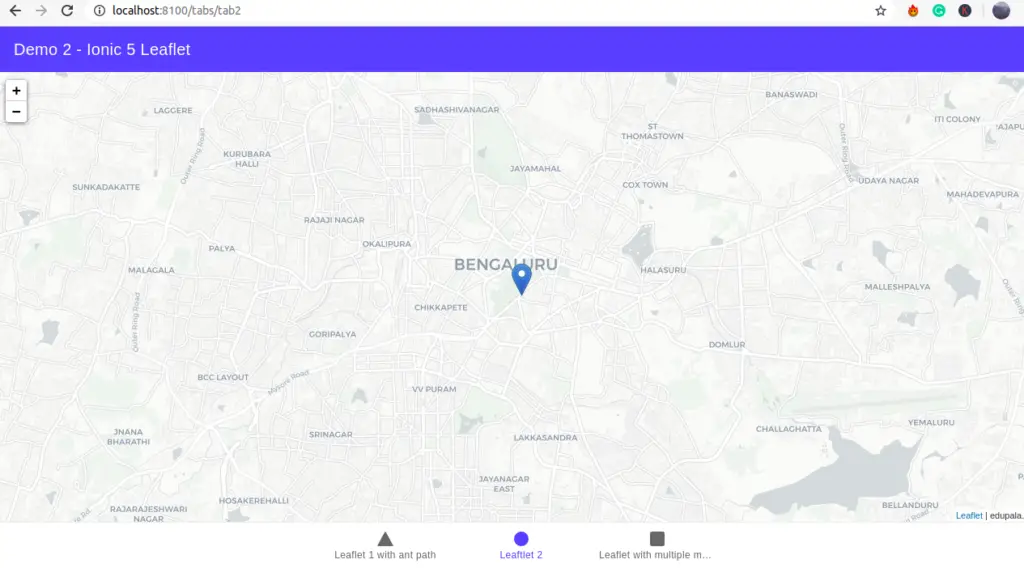
<ion-header [translucent]="true">
<ion-toolbar color="primary">
<ion-title>
Demo 2 - Ionic 5 Leaflet
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content [fullscreen]="true">
<div id="mapId2" style="width: 100%; height: 100%"></div>
</ion-content>
import { Component } from '@angular/core';
import * as Leaflet from 'leaflet';
@Component({
selector: 'app-tab2',
templateUrl: 'tab2.page.html',
styleUrls: ['tab2.page.scss']
})
export class Tab2Page {
map: Leaflet.Map;
ionViewDidEnter() {
this.leafletMap();
}
leafletMap() {
this.map = new Leaflet.Map('mapId2').setView([12.972442, 77.594563], 13);
Leaflet.tileLayer('http://{s}.basemaps.cartocdn.com/light_all/{z}/{x}/{y}.png', {
attribution: 'edupala.com'
}).addTo(this.map);
const markPoint = Leaflet.marker([12.972442, 77.594563]);
markPoint.bindPopup('<p>Tashi Delek - Bangalore.</p>');
this.map.addLayer(markPoint);
}
ionViewWillLeave() {
this.map.remove();
}
}
Step 5: Adding multiple markers in Leaflet Map
In tab1 and tab2 we display a leaflet map with a single marker. In tab3 we will add multiple leaflet markers in the Leaflet map.
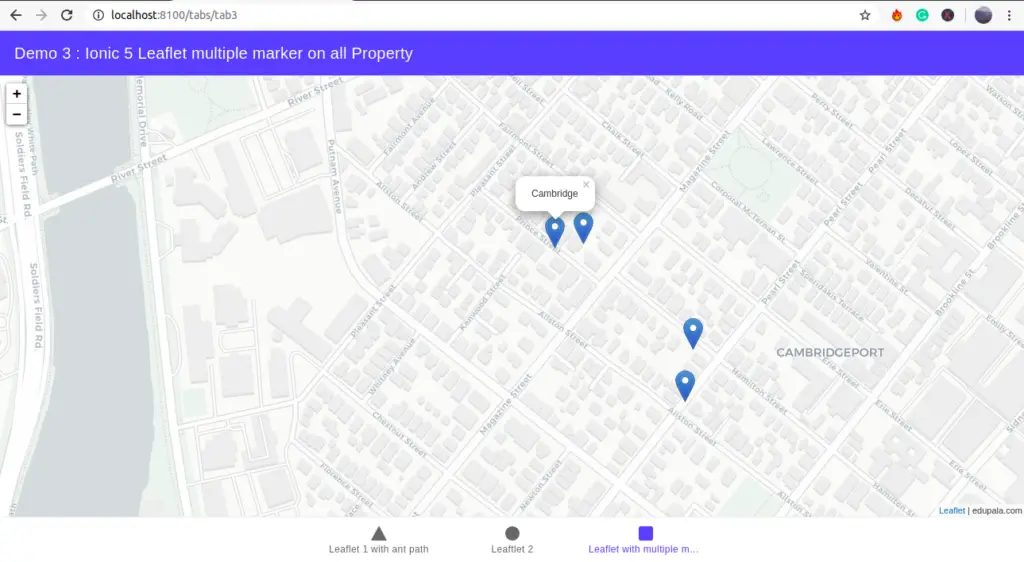
Add the following data on data.JSON in www/assets/data.json as
{
"properties": [
{
"city": "Cambridge",
"state": "MA",
"long": -71.10858,
"lat": 42.35963
},
{
"city": "Cambridge",
"state": "MA",
"long": -71.10869,
"lat": 42.359103
},
{
"city": "Boston",
"state": "MA",
"long": -71.110061,
"lat": 42.360686
},
{
"city": "Cambridge",
"long": -71.110448,
"lat": 42.360642
}
]
}
Edit tab3.page.html
<ion-header [translucent]="true">
<ion-toolbar color="primary">
<ion-title>
Demo 3 : Ionic 5 Leaflet multiple marker on all Property
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content [fullscreen]="true">
<div id="mapId3" style="width: 100%; height: 100%"></div>
</ion-content>
import { Component } from '@angular/core';
import * as Leaflet from 'leaflet';
@Component({
selector: 'app-tab3',
templateUrl: 'tab3.page.html',
styleUrls: ['tab3.page.scss']
})
export class Tab3Page {
map: Leaflet.Map;
propertyList = [];
constructor() { }
ionViewDidEnter() {
this.map = new Leaflet.Map('mapId3').setView([42.35663, -71.1109], 16);
Leaflet.tileLayer('http://{s}.basemaps.cartocdn.com/light_all/{z}/{x}/{y}.png', {
attribution: 'edupala.com'
}).addTo(this.map);
fetch('./assets/data.json')
.then(res => res.json())
.then(data => {
this.propertyList = data.properties;
this.leafletMap();
})
.catch(err => console.error(err));
}
leafletMap() {
for (const property of this.propertyList) {
Leaflet.marker([property.lat, property.long]).addTo(this.map)
.bindPopup(property.city)
.openPopup();
}
}
ionViewWillLeave() {
this.map.remove();
}
}
Conclusion
In this tutorial, we have completed our three examples of leaflet maps in the Ionic project. There are many open-source javascript libraries to include maps in our project. The Leaflet holds the leading position among open-source JavaScript libraries for interactive maps.
Related posts
- How to use Highcharts in Ionic?
- How to add Ionic charts and graphs in Ionic?
- How to use D3js in Ionic 5?
- How to use the ionic skeleton component.
- How to create a different ionic list.
Hi Edu, thank you very much for this tutorial. Unfortunately, I get an error when I try Step 5: Adding multiple markers in Leaflet Map.
Inside the leafletMap() function, the properties lat, long and city don’t exist on type “never”.
I only need this AllMap-Component, because I need multiple markers. Are there any dependencies? Do you what could be wrong?
Thank you in advance. BB
Hi Ben, can you please check this leaflet multiple markers ionic ups on GitHub. I had uploaded just now so that you can refer it. I hope it will help you. I made this app a few months back, I don’t remember where I face the problem. This site is my personal note. If this blog helps you and others, I feel glad. here is GitHub link https://github.com/ngodup/ionicLeaflet
Hi Edu, thank you for your quick response and the provision of the repo.
I face a problem in the allMap.ts. I cannot access the keys (properties) of the data.json file, the error is called ‘Property ‘lat’ does not exist on type ‘never’.’ Same goes for the long and the city. In addition, in line 14, I receive the error ‘Member ‘map’ implicitly has an ‘any’ type.’
I am using VS Code and Ionic 3 and have a running project. The Map is a component I want to use.
Hi Ben, If you have copied the data.json from my site. If so then there is extra colon at {
“properties” : [
{,
Just remove is a colon, I guess it because of this you’re getting an error.
Hi, thank you for your tutorial. I have an issue with my leaflep marker, to be more specific what I’m trying to do is to create a clickable word inside popup content (some work like: Click) then when someone clicks on the word this push to one of my pages inside app, like HomePage.
Hi Edu thank you for your tutorial, It worked.
But I’trying to advance and I’m trying to add function locate.
In my browser PC it’s working, but when I open it in mobile browser it doesen’t show the locate.
I’m not understanding.
When I open an example of Leaflet page from my phone It work. The browser ask me about locate authorization and work, but in my application, the browser doesn’t ask me authorization and doesn’t show me the locate.
Do you have another tutorial to help me?
Or do you have any idea about this problem?
My Ionic version is 6.20.1 and Leaflet 1.9.2.
Thany you for your attention.