We have seen popular eCommerce companies like Amazon provide a facility for adding signatures on a device when we receive our order. In angular, we can easily implement this feature in our application using third-party libraries. We can also add signatures without using any third party also.
In this tutorial, we have demonstrated how to add an angular signature pad to our application. We have demonstrated two examples of angular signature capture using Javascript signature_pad and angular2-signaturepad libraries.
Setting up and configuring Angular signature pad project
Let’s first create our Angular signature pad project and we have two examples of angular signature capture.
ng new signatureApp
Here is a screenshot of our first example of an Angular signature pad using the angular2-signaturepad library.
Angular signature Pad example 1 using angular2-signaturepad library
Let’s install our angular2-signaturepad library in our angular project first.
npm install angular2-signaturepad --save
To use this angular signature capture we have to import the angular2-signaturepad library in our app.module.ts file and let import it.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { SignaturePadModule } from 'angular2-signaturepad';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
SignaturePadModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Edit the app.component.html template to add the signature component tag and it has some events which are raised while drawing the signature on the signature component.
We also have two buttons one to clear the signature pad and the other to save in a variable signatureImg which we used to display the signature image in our template. Clicking on Save signature will save the signature pad in image or base64 and we can display its signature image using img element.
<h4>{{ title }}</h4>
<div class="signature-container">
<signature-pad [options]="signaturePadOptions" (onBeginEvent)="drawStart()" (onEndEvent)="drawComplete()"></signature-pad>
</div>
<div class="buttons">
<button (click)="clearSignature()">Clear signature pad</button>
<button (click)="savePad()">Save signature</button>
</div>
<div class="signature-image" *ngIf="signatureImg">
<img src='{{ signatureImg }}' />
</div>
<router-outlet></router-outlet>
When we capture a signature, its data are saved in base64 string format, this allows us to save signature data in the database, and we can also edit or modify the signature easily.
In our typescript component file, we have added code to configure the Angular signature pad like the width, height, and thickness of the pen drawing. Let edit the app.component.ts file
import { Component, ViewChild } from '@angular/core';
import { SignaturePad } from 'angular2-signaturepad';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
title = 'Angular signature example';
signatureImg: string;
@ViewChild(SignaturePad) signaturePad: SignaturePad;
signaturePadOptions: Object = {
'minWidth': 2,
'canvasWidth': 700,
'canvasHeight': 300
};
constructor() { }
ngAfterViewInit() {
// this.signaturePad is now available
this.signaturePad.set('minWidth', 2);
this.signaturePad.clear();
}
drawComplete() {
console.log(this.signaturePad.toDataURL());
}
drawStart() {
console.log('begin drawing');
}
clearSignature() {
this.signaturePad.clear();
}
savePad() {
const base64Data = this.signaturePad.toDataURL();
this.signatureImg = base64Data;
}
}
We have also added a few styles to our signature container and button. Let’s edit the app.component.scss file.
.signature-container {
border-style: dashed;
border-width: 1px;
margin-bottom: 20px;
background-color: coral;
}
.buttons {
float: right;
button {
margin-right: 10px;
padding: 10px;
background-color: dodgerblue;
color: white;
border-radius: 5px;
}
}
Angular signature Pad example 2 using signature_pad Javascript library
In our second example, we will use the signature_pad Javascript library to implement the Angular signature pad example. Let’s first install the signature_pad library in our project.
npm i signature_pad --save
In our app.component.html template, we need to add a canvas to draw our signature. We have two buttons one to clear the signature canvas and the other to get signature data in base64 string format.
We have to define a canvas element where we are writing our signature on it. Once we have our signature in base64 we can display it as an image using the IMG element in our template.
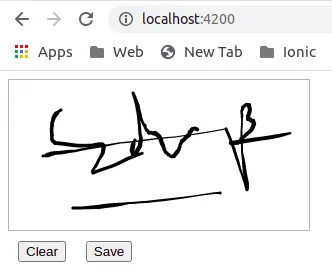
<canvas #canvas (touchstart)="startDrawing($event)" (touchmove)="moved($event)"></canvas>
<button (click)="clearPad()">Clear</button>
<button color="secondary" (click)="savePad()">Save</button>
<img src='{{ signatureImg }}' />
Edit app.component.ts typescript file code to draw a signature on canvas and save in base64 formats.
import { Component, ElementRef, ViewChild } from '@angular/core';
import SignaturePad from 'signature_pad';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
title = 'signatureJS';
signaturePad: SignaturePad;
@ViewChild('canvas') canvasEl: ElementRef;
signatureImg: string;
constructor() { }
ngAfterViewInit() {
this.signaturePad = new SignaturePad(this.canvasEl.nativeElement);
}
startDrawing(event: Event) {
console.log(event);
// works in device not in browser
}
moved(event: Event) {
// works in device not in browser
}
clearPad() {
this.signaturePad.clear();
}
savePad() {
const base64Data = this.signaturePad.toDataURL();
this.signatureImg = base64Data;
}
}
Once we have our canvas element add style like border, background, and more on a canvas element. Let’s edit the app.component.scss file.
canvas {
display: block;
border: 1px solid rgb(187, 178, 178);
background-color: var(--ion-color-success);
}
button {
margin: 10px;
margin-left: 10px;
}
Conclusion
We have completed two examples of drawing and capturing signatures in our Angular application using two different third-party libraries angular2-signaturepad and Javascript signature_pad library. All signature data are saved in base64 format and we can easily display it in our template using the img tag and we can convert base64 to an image file also.
Related posts
- How to calculate base64 image size in Angular
- How to install Angular material
- How to implement CKEditor in Angular
- How to use to implement HighCharts Angular
Is there any way to load base64 image in signaturepad.
i want to load signature which i save earlier
Once you had saved the image in base64 format, we can assign it directly to image src it will display as image
Thanks so much for this